js创建和读取对象多层属性的方法总结
2023-08-12
js复杂对象存在深层嵌套的属性,在实际项目中往往需要精准创建和读取多层属性,本文总结一些常用的方法。
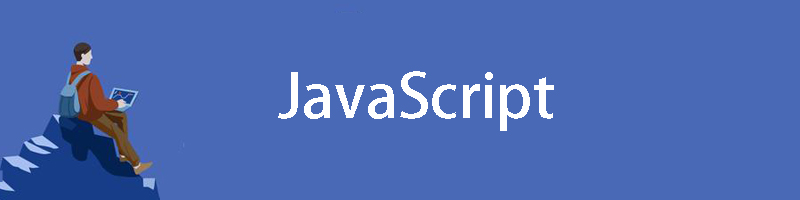
一.创建对象深层属性
/**
* 设置对象深层属性
*/
const setDeepValue = (object, path, value) => {
let fieldPath = [...path];
if (fieldPath.length) {
const key = fieldPath.shift();
object = object || {};
object[key] = setDeepValue(object[key], fieldPath, value);
}
else {
object = value;
}
return object;
};
参考资料:https://blog.csdn.net/weixin_44636596/article/details/118553109
二.读取对象深层属性
通常有三种方法来实现。
1.析构
const person = {
name: 'John',
age: 30,
address: {
street: '123 Main St',
city: 'Anytown',
state: 'CA',
zip: '12345'
}
};
如果我们想要访问address对象中的属性,我们可以使用对象多层解构:
const { name, age, address: { street, city, state, zip } } = person;
参考资料:https://juejin.cn/post/7244818201975799866
2.可选链运算符(?.)
资料:https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Operators/Optional_chaining
非常好用,但是兼容性差,毕竟是新属性。
const adventurer = {
name: 'Alice',
cat: {
name: 'Dinah',
},
};
const dogName = adventurer.dog?.name;
console.log(dogName);
// Expected output: undefined
console.log(adventurer.someNonExistentMethod?.());
// Expected output: undefined
3.递归读取
自定义逻辑读取属性,能达到目的就行,方法千千万。
这里推荐参考使用开源的第三方库:get-object-field
var gof = function(obj, def, replace) {
var getValue = function(field) {
if (field !== undefined) {
// 字段值为 undefined 或 null 都会使用默认值
if (obj && obj[field] !== undefined && obj[field] !== null) {
obj = obj[field];
} else {
obj = def;
}
return getValue;
}
if (replace !== undefined && obj === replace) {
return def;
}
return obj;
};
getValue.toString = function() {
return obj;
};
getValue.valueOf = function() {
return obj;
};
return getValue;
}